Table Of Contents
Screen Manager(翻訳中)¶
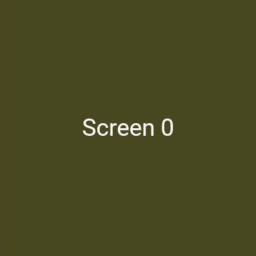
バージョン 1.4.0 で追加.
スクリーンマネージャは、アプリケーションの複数の画面を管理するためのwidgetです。 デフォルトの ScreenManager
は一度に1つの Screen
のみを表示し、 TransitionBase
を使用して1つの画面から別の画面に切り替わります。
画面の座標/スケールを変更したり、カスタムシェイダーを使用して派手なアニメーションを実行したりすることによって複数のトランジションがサポートされます。
Basic Usage(基本的な使い方)¶
4つの名前のスクリーンでスクリーン・マネージャを構築してみましょう。 画面を作成するときは、必ず名前を付ける必要があります
from kivy.uix.screenmanager import ScreenManager, Screen
# Create the manager
sm = ScreenManager()
# Add few screens
for i in range(4):
screen = Screen(name='Title %d' % i)
sm.add_widget(screen)
# By default, the first screen added into the ScreenManager will be
# displayed. You can then change to another screen.
# Let's display the screen named 'Title 2'
# A transition will automatically be used.
sm.current = 'Title 2'
ScreenManager.transition
のデフォルトは、direction
は duration
がオプションの SlideTransition
です。
デフォルトでは、 Screen
には何も表示されません。これは単なる RelativeLayout
です。 そのクラスを独自のスクリーンのroot widget として使用する必要があります。サブクラス化するのが最善の方法です。
警告
Screen
は RelativeLayout
であるため、Common Pitfalls を理解することが重要です。
‘Menu Screen’ と’ Settings Screen’ の例を以下に示します:
from kivy.app import App
from kivy.lang import Builder
from kivy.uix.screenmanager import ScreenManager, Screen
# Create both screens. Please note the root.manager.current: this is how
# you can control the ScreenManager from kv. Each screen has by default a
# property manager that gives you the instance of the ScreenManager used.
Builder.load_string("""
<MenuScreen>:
BoxLayout:
Button:
text: 'Goto settings'
on_press: root.manager.current = 'settings'
Button:
text: 'Quit'
<SettingsScreen>:
BoxLayout:
Button:
text: 'My settings button'
Button:
text: 'Back to menu'
on_press: root.manager.current = 'menu'
""")
# Declare both screens
class MenuScreen(Screen):
pass
class SettingsScreen(Screen):
pass
# Create the screen manager
sm = ScreenManager()
sm.add_widget(MenuScreen(name='menu'))
sm.add_widget(SettingsScreen(name='settings'))
class TestApp(App):
def build(self):
return sm
if __name__ == '__main__':
TestApp().run()
Changing Direction(変化する方向)¶
ScreenManager
の一般的な使用例では、次の画面にスライドして前の画面にスライドするSlideTransitionを使用します。 前の例を基にして、これは次のように行うことができます:
Builder.load_string("""
<MenuScreen>:
BoxLayout:
Button:
text: 'Goto settings'
on_press:
root.manager.transition.direction = 'left'
root.manager.current = 'settings'
Button:
text: 'Quit'
<SettingScreen>:
BoxLayout:
Button:
text: 'My settings button'
Button:
text: 'Back to menu'
on_press:
root.manager.transition.direction = 'right'
root.manager.current = 'menu'
""")
Advanced Usage(応用例)¶
1.8.0から、 switch_to()
を使用して、動的に新しい画面に切り替えたり、遷移オプションを変更したり、前の画面を削除したりすることができます。
sm = ScreenManager()
screens = [Screen(name='Title {}'.format(i)) for i in range(4)]
sm.switch_to(screens[0])
# later
sm.switch_to(screens[1], direction='right')
このメソッドは ScreenManager
インスタンスに画面を追加するので、画面がすでにこのインスタンスに追加されている場合は使用しないでください。 すでに追加されている画面に切り替えるには、 current
プロパティを使用する必要があります。
Changing transitions(トラジッションの変更)¶
デフォルトでは次のような複数のトランジションが使用できます。
NoTransition
- アニメーションなしで瞬時に画面を切り替えるSlideTransition
- 任意の方向から画面を出し入れするCardTransition
- モードに応じて、前のスライドまたは新しいスライドを新しいものからスライドさせるSwapTransition
- iOSスワップトランジッションの実装FadeTransition
- スクリーンのフェードイン/アウトを行うシェーダWipeTransition
- スクリーンを右から左に拭くためのシェーダFallOutTransition
- 古いスクリーンが ‘落ちて’ 透明になるシェーダ。背後に新しいスクリーンが現れます。RiseInTransition
- 透明から不透明にフェードしながら新しい画面が画面中心から浮かび上がるシェーダ。
ScreenManager.transitionプロパティを変更することで簡単にトランジションを切り替えれます:
sm = ScreenManager(transition=FadeTransition())
注釈
現在、Shaderベースのトランジションのいずれもアンチエイリアスを使用していません。 これは、スーパーサンプリングを処理するロジックを持たないFBOを使用するためです。 これは既知の問題であり画面上にレンダリングされた場合と同じ結果をもたらす透過的な実装に取り組んでいます。
より具体的には、アニメーション中にシャープなエッジのテキストが見える場合は、それは正常です。
-
class
kivy.uix.screenmanager.
Screen
(**kw)[ソース]¶ ベースクラス:
kivy.uix.relativelayout.RelativeLayout
Screenは
ScreenManager
で使用するための要素です。 詳細については、モジュールのマニュアルを参照してください。Events: - on_pre_enter: ()
画面が使用されようとしたときに発生するイベント:入力アニメーションが開始されます。
- on_enter: ()
画面が表示されたときに起動されるイベント:入力アニメーションが完了した。
- on_pre_leave: ()
画面が削除されようとしたときに発生するイベント:離脱アニメーションが開始されます。
- on_leave: ()
画面が削除されると発生するイベント:終了アニメーションが終了しました。
バージョン 1.6.0 で変更: Events on_pre_enter, on_enter, on_pre_leave and on_leave were added.
-
manager
¶ ScreenManager
オブジェクト。画面がマネージャに追加されたときに設定されます。manager
はObjectProperty
であり、デフォルトはNone(読み取り専用)です。
-
name
¶ ScreenManager
内で一意でなければならない画面の名前。 これは:attr:ScreenManager.current で使用される名前です。name
はStringProperty
でデフォルトは”“です。
-
transition_progress
¶ Value that represents the completion of the current transition, if any is occurring.
トランジションが進行中であれば、モードにかかわらず、値は0から1に変わります。トランジションかトランジションかを知りたい場合は
transition_state
を確認してください。transition_progress
はNumericProperty
で、デフォルトは0です。
-
transition_state
¶ 遷移の状態を表す値:
移行が画面を表示する場合は ‘in’
遷移があなたの画面を隠す場合は ‘out’
遷移が完了すると、状態は最後の値(インまたはアウト)を保持します。
transition_state
はOptionProperty
で、デフォルトは ‘out’ です。
-
class
kivy.uix.screenmanager.
ScreenManager
(**kwargs)[ソース]¶ ベースクラス:
kivy.uix.floatlayout.FloatLayout
スクリーンマネージャー。 これは、
Screen
のスタックとメモリを制御するメインクラスです。By default, the manager will show only one screen at a time.
-
current
¶ Name of the screen currently shown, or the screen to show.
from kivy.uix.screenmanager import ScreenManager, Screen sm = ScreenManager() sm.add_widget(Screen(name='first')) sm.add_widget(Screen(name='second')) # By default, the first added screen will be shown. If you want to # show another one, just set the 'current' property. sm.current = 'second'
current
is aStringProperty
and defaults to None.
-
current_screen
¶ Contains the currently displayed screen. You must not change this property manually, use
current
instead.current_screen
is anObjectProperty
and defaults to None, read-only.
-
get_screen
(name)[ソース]¶ Return the screen widget associated with the name or raise a
ScreenManagerException
if not found.
-
screen_names
¶ List of the names of all the
Screen
widgets added. The list is read only.screens_names
is anAliasProperty
and is read-only. It is updated if the screen list changes or the name of a screen changes.
-
screens
¶ List of all the
Screen
widgets added. You should not change this list manually. Use theadd_widget
method instead.screens
is aListProperty
and defaults to [], read-only.
-
switch_to
(screen, **options)[ソース]¶ Add a new screen to the ScreenManager and switch to it. The previous screen will be removed from the children. options are the
transition
options that will be changed before the animation happens.If no previous screens are available, the screen will be used as the main one:
sm = ScreenManager() sm.switch_to(screen1) # later sm.switch_to(screen2, direction='left') # later sm.switch_to(screen3, direction='right', duration=1.)
If any animation is in progress, it will be stopped and replaced by this one: you should avoid this because the animation will just look weird. Use either
switch_to()
orcurrent
but not both.The screen name will be changed if there is any conflict with the current screen.
-
transition
¶ Transition object to use for animating the transition from the current screen to the next one being shown.
For example, if you want to use a
WipeTransition
between slides:from kivy.uix.screenmanager import ScreenManager, Screen, WipeTransition sm = ScreenManager(transition=WipeTransition()) sm.add_widget(Screen(name='first')) sm.add_widget(Screen(name='second')) # by default, the first added screen will be shown. If you want to # show another one, just set the 'current' property. sm.current = 'second'
transition
is anObjectProperty
and defaults to aSlideTransition
.バージョン 1.8.0 で変更: Default transition has been changed from
SwapTransition
toSlideTransition
.
-
-
exception
kivy.uix.screenmanager.
ScreenManagerException
[ソース]¶ ベースクラス:
Exception
Exception for the
ScreenManager
.
-
class
kivy.uix.screenmanager.
TransitionBase
[ソース]¶ ベースクラス:
kivy.event.EventDispatcher
TransitionBase is used to animate 2 screens within the
ScreenManager
. This class acts as a base for other implementations like theSlideTransition
andSwapTransition
.Events: - on_progress: Transition object, progression float
Fired during the animation of the transition.
- on_complete: Transition object
Fired when the transition is finished.
-
add_screen
(screen)[ソース]¶ (internal) Used to add a screen to the
ScreenManager
.
-
duration
¶ Duration in seconds of the transition.
duration
is aNumericProperty
and defaults to .4 (= 400ms).バージョン 1.8.0 で変更: Default duration has been changed from 700ms to 400ms.
-
is_active
¶ Indicate whether the transition is currently active or not.
is_active
is aBooleanProperty
and defaults to False, read-only.
-
manager
¶ ScreenManager
オブジェクト。画面がマネージャに追加されたときに設定されます。manager
はObjectProperty
であり、デフォルトはNone(読み取り専用)です。
-
remove_screen
(screen)[ソース]¶ (internal) Used to remove a screen from the
ScreenManager
.
-
screen_in
¶ Property that contains the screen to show. Automatically set by the
ScreenManager
.screen_in
is anObjectProperty
and defaults to None.
-
screen_out
¶ Property that contains the screen to hide. Automatically set by the
ScreenManager
.screen_out
is anObjectProperty
and defaults to None.
-
start
(manager)[ソース]¶ (internal) Starts the transition. This is automatically called by the
ScreenManager
.
-
stop
()[ソース]¶ (internal) Stops the transition. This is automatically called by the
ScreenManager
.
-
class
kivy.uix.screenmanager.
ShaderTransition
[ソース]¶ ベースクラス:
kivy.uix.screenmanager.TransitionBase
Transition class that uses a Shader for animating the transition between 2 screens. By default, this class doesn’t assign any fragment/vertex shader. If you want to create your own fragment shader for the transition, you need to declare the header yourself and include the “t”, “tex_in” and “tex_out” uniform:
# Create your own transition. This shader implements a "fading" # transition. fs = """$HEADER uniform float t; uniform sampler2D tex_in; uniform sampler2D tex_out; void main(void) { vec4 cin = texture2D(tex_in, tex_coord0); vec4 cout = texture2D(tex_out, tex_coord0); gl_FragColor = mix(cout, cin, t); } """ # And create your transition tr = ShaderTransition(fs=fs) sm = ScreenManager(transition=tr)
-
clearcolor
¶ Sets the color of Fbo ClearColor.
バージョン 1.9.0 で追加.
clearcolor
is aListProperty
and defaults to [0, 0, 0, 1].
-
fs
¶ Fragment shader to use.
fs
is aStringProperty
and defaults to None.
-
vs
¶ Vertex shader to use.
vs
is aStringProperty
and defaults to None.
-
-
class
kivy.uix.screenmanager.
SlideTransition
[ソース]¶ ベースクラス:
kivy.uix.screenmanager.TransitionBase
Slide Transition, can be used to show a new screen from any direction: left, right, up or down.
-
direction
¶ Direction of the transition.
direction
is anOptionProperty
and defaults to ‘left’. Can be one of ‘left’, ‘right’, ‘up’ or ‘down’.
-
-
class
kivy.uix.screenmanager.
SwapTransition
(**kwargs)[ソース]¶ ベースクラス:
kivy.uix.screenmanager.TransitionBase
Swap transition that looks like iOS transition when a new window appears on the screen.
-
class
kivy.uix.screenmanager.
FadeTransition
[ソース]¶ ベースクラス:
kivy.uix.screenmanager.ShaderTransition
Fade transition, based on a fragment Shader.
-
class
kivy.uix.screenmanager.
WipeTransition
[ソース]¶ ベースクラス:
kivy.uix.screenmanager.ShaderTransition
Wipe transition, based on a fragment Shader.
-
class
kivy.uix.screenmanager.
FallOutTransition
[ソース]¶ ベースクラス:
kivy.uix.screenmanager.ShaderTransition
Transition where the new screen ‘falls’ from the screen centre, becoming smaller and more transparent until it disappears, and revealing the new screen behind it. Mimics the popular/standard Android transition.
バージョン 1.8.0 で追加.
-
duration
¶ Duration in seconds of the transition, replacing the default of
TransitionBase
.duration
is aNumericProperty
and defaults to .15 (= 150ms).
-
-
class
kivy.uix.screenmanager.
RiseInTransition
[ソース]¶ ベースクラス:
kivy.uix.screenmanager.ShaderTransition
Transition where the new screen rises from the screen centre, becoming larger and changing from transparent to opaque until it fills the screen. Mimics the popular/standard Android transition.
バージョン 1.8.0 で追加.
-
duration
¶ Duration in seconds of the transition, replacing the default of
TransitionBase
.duration
is aNumericProperty
and defaults to .2 (= 200ms).
-
-
class
kivy.uix.screenmanager.
NoTransition
[ソース]¶ ベースクラス:
kivy.uix.screenmanager.TransitionBase
No transition, instantly switches to the next screen with no delay or animation.
バージョン 1.8.0 で追加.
-
class
kivy.uix.screenmanager.
CardTransition
[ソース]¶ ベースクラス:
kivy.uix.screenmanager.SlideTransition
Card transition that looks similar to Android 4.x application drawer interface animation.
It supports 4 directions like SlideTransition: left, right, up and down, and two modes, pop and push. If push mode is activated, the previous screen does not move, and the new one slides in from the given direction. If the pop mode is activated, the previous screen slides out, when the new screen is already on the position of the ScreenManager.
バージョン 1.10 で追加.
-
mode
¶ Indicates if the transition should push or pop the screen on/off the ScreenManager.
- ‘push’ means the screen slides in in the given direction
- ‘pop’ means the screen slides out in the given direction
mode
is anOptionProperty
and defaults to ‘push’.
-
start
(manager)[ソース]¶ (internal) Starts the transition. This is automatically called by the
ScreenManager
.
-