Table Of Contents
Box Layout¶
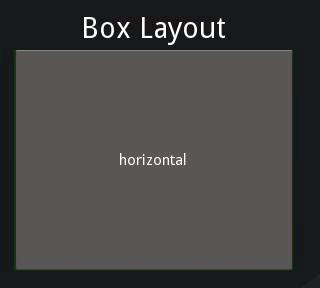
BoxLayout
arranges children in a vertical or horizontal box.
To position widgets above/below each other, use a vertical BoxLayout:
layout = BoxLayout(orientation='vertical')
btn1 = Button(text='Hello')
btn2 = Button(text='World')
layout.add_widget(btn1)
layout.add_widget(btn2)
To position widgets next to each other, use a horizontal BoxLayout. In this example, we use 10 pixel spacing between children; the first button covers 70% of the horizontal space, the second covers 30%:
layout = BoxLayout(spacing=10)
btn1 = Button(text='Hello', size_hint=(.7, 1))
btn2 = Button(text='World', size_hint=(.3, 1))
layout.add_widget(btn1)
layout.add_widget(btn2)
Position hints are partially working, depending on the orientation:
- If the orientation is vertical: x, right and center_x will be used.
- If the orientation is horizontal: y, top and center_y will be used.
You can check the examples/widgets/boxlayout_poshint.py for a live example.
注釈
The size_hint uses the available space after subtracting all the fixed-size widgets. For example, if you have a layout that is 800px wide, and add three buttons like this:
btn1 = Button(text='Hello', size=(200, 100), size_hint=(None, None))
btn2 = Button(text='Kivy', size_hint=(.5, 1))
btn3 = Button(text='World', size_hint=(.5, 1))
The first button will be 200px wide as specified, the second and third will be 300px each, e.g. (800-200) * 0.5
バージョン 1.4.1 で変更: Added support for pos_hint.
-
class
kivy.uix.boxlayout.
BoxLayout
(**kwargs)[ソース]¶ ベースクラス:
kivy.uix.layout.Layout
Box layout class. See module documentation for more information.
-
minimum_height
¶ Automatically computed minimum height needed to contain all children.
バージョン 1.10.0 で追加.
minimum_height
is aNumericProperty
and defaults to 0. It is read only.
-
minimum_size
¶ Automatically computed minimum size needed to contain all children.
バージョン 1.10.0 で追加.
minimum_size
is aReferenceListProperty
of (minimum_width
,minimum_height
) properties. It is read only.
-
minimum_width
¶ Automatically computed minimum width needed to contain all children.
バージョン 1.10.0 で追加.
minimum_width
is aNumericProperty
and defaults to 0. It is read only.
-
orientation
¶ Orientation of the layout.
orientation
is anOptionProperty
and defaults to ‘horizontal’. Can be ‘vertical’ or ‘horizontal’.
-
padding
¶ Padding between layout box and children: [padding_left, padding_top, padding_right, padding_bottom].
padding also accepts a two argument form [padding_horizontal, padding_vertical] and a one argument form [padding].
バージョン 1.7.0 で変更: Replaced NumericProperty with VariableListProperty.
padding
is aVariableListProperty
and defaults to [0, 0, 0, 0].
-
spacing
¶ Spacing between children, in pixels.
spacing
is aNumericProperty
and defaults to 0.
-